NPTEL Data Structure And Algorithms Using Java Assignment Solutions
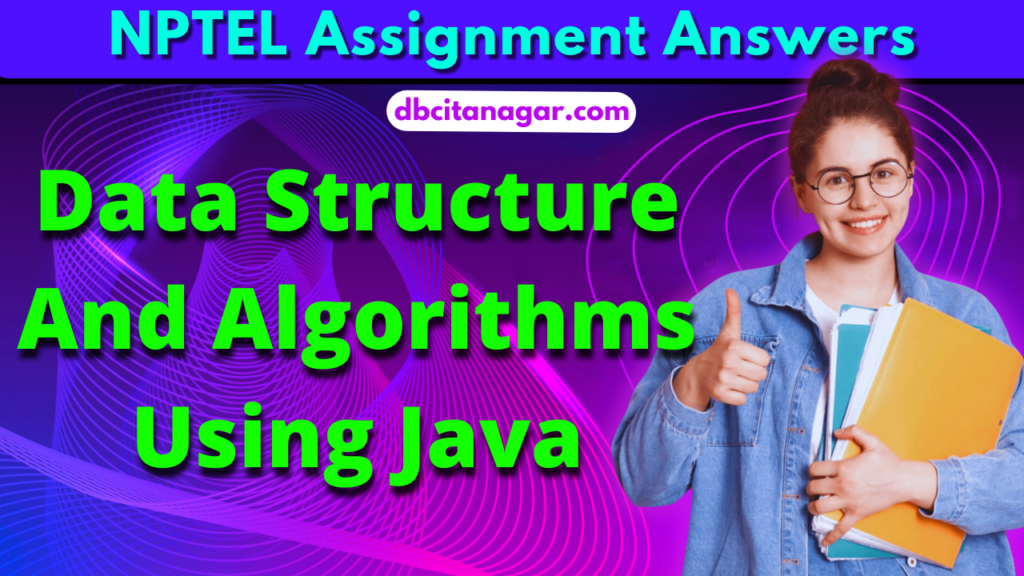
NPTEL Data Structure And Algorithms Using Java Week 3 Assignment Answers 2023
1. Which data structure is the collection of finite, ordered, and homogeneous data elements?
a. Array
b. Graph
c. Мар
d. Trees
Answer :- For Answer Click Here
2. Consider the following piece of code.
public class Main {
public static void main(String args[]) {
char[] a = {'a', 'b', 'c', 'd', 'e', 'f'};
for (int i = 0; i < a.length; i = i + 2)
System.out.print(" " + a[i]);
}
}
Which of the following is an output of the above program?
a. abodef
b. ace
c. bdf
d. abdf
Answer :- For Answer Click Here
3. Consider the following program.
public class Main {
public static void main(String args[]) {
int[] a = new int[0];
a[0] = 12;
System.out.println(a);
}
}
Which of the following is an output of the above program?
a. 12
b. Exception
c. O
d. Null
Answer :-
5. Which of the following statements is true regarding ArrayList in Java?
a. ArrayList is a synchronized data structure, making it thread-safe.
b. ArrayList allows duplicate elements and maintains insertion order.
c. ArrayList is a resizable array that automatically increases its size when elements are added.
d. ArrayList can only store primitive data types like int, double, and char.
Answer :- For Answer Click Here
6. Which of the following classes implement a dynamic array?
a. ArrayList
b. LinkedList
c. DynamicList
d. AbstractList
Answer :-
7. Which method is used to remove all elements from an ArrayList?
a. removeAllO
b. clear
c. deleteAllO
d. eraseA110
Answer :-
8. What will be the output of the following Java code?

Which of the following is an output of the above program?
а. “Apple”
b. “Banana”
c. “Orange”
d. IndexOutOfBoundsException will be thrown.
Answer :- For Answer Click Here
9. What is the time complexity of adding an element at the end of an ArrayList?
a. O(1)
b. O(log n)
c. O(n)
d. O(n log n)
Answer :-
10. Which of the following methods is used to find the index of the first occurrence of a specific element in an ArravList?
a. findIndex
b. indexOf0
c. search
d. findFirstO
Answer :- For Answer Click Here
Course Name | Data Structure And Algorithms Using Java |
Category | NPTEL Assignment Answer |
Home | Click Here |
Join Us on Telegram | Click Here |
NPTEL Data Structure And Algorithms Using Java Week 2 Assignment Answers 2023
1. Which of the following is/are FALSE in the case of Data Structure in Java?
a. Array is an indexed linear data structure
b. linked list is a sequential non- linear data structure
C. stack and queue are Linear data structure
d. set, tree, table, and graph are Non-linear data structure
Answer :- b. linked list is a sequential non- linear data structure
2. Which of the following is True in the case of Java Collection Framework(JCF)?
a. JCF provides mid-performance software encoding
b. Extending and or adapting a collection is easy and not flexible with JCF
c. The JCF is consist of : Collections and Facilities
d. The JCF doesn’t allow different types of collections to work with a high degree of interoperability.
Answer :- c. The JCF is consist of : Collections and Facilities
3. Which among the following is a Java Collection Framework(JCF) Class?
a. Set
b. List
c. Queue
d. Vector
Answer :- d. Vector
4. Choose the right option to complete the following sentence in the case of EnumSet EnumSet extends AbstractSet and implements
a. Enum type
b. Enum
c. AbstractSet
d. Set
Answer :- d. Set
5. Which of the following statement is incorrect with respect to ‘Map’ in Java?
a. A Map is an obiect that maps keys to values
b. Map is a collection of key-value pairs.
c. Map is a generic interface that has the declaration: interface Map <I>
d. SortedMap interface doesn’t extend Map.
Answer :- d. SortedMap interface doesn't extend Map.
6. Which of the following statements is/are incorrect for Java Collection Framework (JCF)?
a. Vector is similar to. ArrayList, which represents a dynamic array
b. Stack is a subclass of Vector that implements a standard last-in. first-out stack.
c. Unlike HashMap. Hashtable doesn’t store Key/value pairs.
d. Class Properties are used to maintain a list of values in which both key and value are String.
Answer :- c. Unlike HashMap. Hashtable doesn't store Key/value pairs.
7. In Java Collection Framework(JCF) Dictionary is ___________?
a. A concrete implementation
b. An interface
c. An abstract class
d. A data structure for storing only integers
Answer :-c. An abstract class
8. Which of the following interface(s) is (are) NOT descendant(s) of java.util.Collection?
a. java.util. SortedMap
b. java.util.Set
c. java.util. SortedSet
d. java.util. Queue
Answer :- a. java.util. SortedMap
9. Which of the following declaration(s) is(are) not correct to instantiate a collection object?
a. List <data-type list1 = new ArrayList():
b. List <data-type list2 = new LinkedList();
c. List <data-type list3 = new Vector():
d. List <data-type list4 = new PriorityQueue() :
Answer :- d. List <data-type list4 = new PriorityQueue :
10. Which of these interface handle sequences?
a. Set
b. Array
c. List
d. Collection
Answer :- c. List
Course Name | Data Structure And Algorithms Using Java |
Category | NPTEL Assignment Answer |
Home | Click Here |
Join Us on Telegram | Click Here |
NPTEL Data Structure And Algorithms Using Java Week 1 Assignment Answers 2023
1. What is “generic programming” in Java?
a. A programming paradigm that focuses on reusability and type safety.
b. A technique to optimize code execution in Java programs.
c. A feature to dynamically modify variable types at runtime.
d. A method to generate random data in Java programs.
Answer :- a. A programming paradigm that focuses on reusability and type safety. "Generic programming" in Java refers to the use of generics, a programming feature introduced in Java 5 (J2SE 5.0). Generics allow classes, interfaces, and methods to be parameterized with types. By using generics, you can create classes and methods that can work with different types while ensuring type safety. This helps to improve code reusability and maintainability by writing more generic and flexible components.
2. Which symbol is used to denote a generic type in Java?
a. *
b. &
c. #
d. <>
Answer :- d. <> The symbol used to denote a generic type in Java is <>. It is referred to as the diamond operator or angle brackets. Generics in Java allow you to create classes, interfaces, and methods that can work with different types while providing type safety. The diamond operator is used when defining a generic class or when instantiating an object of a generic class to specify the type parameter.
3. Consider the following piece of code.
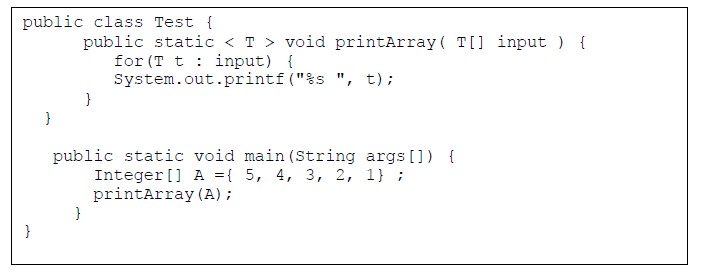
Which of the following is true?
a. The code suffers from syntax errors.
b. Program will give a compile-time error.
c. It will produce output: 5 4 3 2 1
d. Program will yield a run-time error.
Answer :- c. It will produce output: 5 4 3 2 1 The code is correct syntactically and will execute without any compilation errors or runtime errors. It starts the loop with i initialized to 5, and then decrements i in each iteration until it becomes 0 (excluding 0). Therefore, it will print the numbers 5, 4, 3, 2, and 1, each on a separate line.
4. What will be the output of the following code snippet?
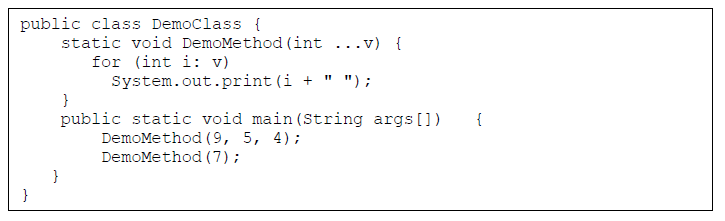
a. Compile-time eifor
b. 9 5 47
c. Run-time error
d. 9 5 4 7
Answer :- d. 9 5 4 7
5.Which of these is wildcard symbol?
a. ?
b. !
C. &
d. %
Answer :- a. Compile-time eifor The option "Compile-time eifor" suggests that there is a typo in the code, and there may be a misspelling of the for keyword. If the code snippet contains "eifor" instead of "for," it will result in a compilation error.
6. Which of the following keywords is used to declare an upper bounded wildcard?
a. bound
b. extends
c. implement
d. super
Answer :- b. extends In Java, the keyword "extends" is used to declare an upper bounded wildcard when working with generics. An upper bounded wildcard restricts the type that can be used as a parameter to a specific class or its subclasses.
7. Can primitive types be used as type arguments in Java generics?
a. Yes, any primitive type can be used.
b. No. only reference types are allowed as type arguments.
c. Yes, but they need to be wrapped in their corresponding wrapper classes.
d. No, primitive types are not compatible with Java generics.
Answer :- c. Yes, but they need to be wrapped in their corresponding wrapper classes.
8. What is type erasure in Java generics?
a. The process of automatically converting generic types to their raw types during compilation.
b. The ability to perform runtime type checking of generic types.
c. The process of inferring type arguments based on method calls.
d. The feature that allows dynamic dispatch of the generic method.
Answer :- a. The process of automatically converting generic types to their raw types during compilation. Type erasure in Java generics is the process by which the compiler removes or "erases" the type parameters used in generic code during compilation. This means that the generic type information is not retained at runtime. Instead, the generic types are replaced with their raw types (non-generic types), which are used for compatibility with pre-generic Java code.
9. Which of the following statements) is(are) true with respect to the use of generics?
a. The Java compiler enforces tighter type checks on generic code at compile time.
b. Generics support programming types as parameters.
c. Generics enable you to implement generic algorithms.
d. In case of a generic method, the same code can work for different types of data.
Answer :- a. The Java compiler enforces tighter type checks on generic code at compile time. b. Generics support programming types as parameters. c. Generics enable you to implement generic algorithms. d. In the case of a generic method, the same code can work for different types of data.
10.

Which of the following statements about the code snippet is correct?
a. The code will compile and execute without any error.
b. code will compile but throw a runtime exception.
c. The code will not compile due to a type mismatch error
d. The code will not compile due to a missing implementation error.
Answer :- c. The code will not compile due to a type mismatch error
Course Name | Data Structure And Algorithms Using Java |
Category | NPTEL Assignment Answer |
Home | Click Here |
Join Us on Telegram | Click Here |